In today’s data-driven world, SQL (Structured Query Language) plays a crucial role in managing and manipulating data. Almost every organisation relies on databases to store and retrieve data, making SQL one of the most valuable skills for anyone pursuing a career in data-related fields. Whether you’re applying for a role as a data analyst, database administrator, or software developer, proficiency in SQL is often a key requirement.
This article aims to provide a comprehensive guide to help you prepare for SQL coding interviews. We will cover the fundamentals of SQL, delve into common interview questions, and offer tips on how to approach these questions effectively. By the end of this article, you should have a solid understanding of SQL and feel more confident as you prepare for your interview.
Understanding SQL Fundamentals for the Interview
Here, we will cover the essential SQL concepts you need to know for your interview. We will discuss topics like databases, tables, queries, and joins. Knowing these fundamentals will give you a solid base for answering questions.
What is SQL?
SQL, or Structured Query Language, is a standardised language used to interact with databases. It allows you to create, read, update, and delete data stored in a relational database. SQL is essential for managing large volumes of data and is widely used in various industries, including finance, healthcare, and technology.
Basic SQL Commands
To effectively prepare for an SQL coding interview, it’s important to understand the basic SQL commands. These commands form the foundation of SQL and are used to perform various operations on data.
SELECT: The SELECT command is used to retrieve data from one or more tables. It allows you to specify the columns you want to retrieve and apply conditions to filter the data.
sql
SELECT column1, column2
FROM table_name;
FROM: The FROM clause specifies the table from which to retrieve the data.
sql
SELECT column1, column2
FROM employees;
WHERE: The WHERE clause is used to filter the data based on specific conditions. It allows you to retrieve only the rows that meet the specified criteria.
sql
SELECT *
FROM employees
WHERE department = ‘Sales’;
ORDER BY: The ORDER BY clause is used to sort the result set based on one or more columns. You can sort the data in ascending (ASC) or descending (DESC) order.
sql
SELECT *
FROM employees
ORDER BY salary DESC;
GROUP BY: The GROUP BY clause is used to group rows that have the same values in specified columns. It is often used with aggregate functions.
sql
SELECT department, COUNT(*)
FROM employees
GROUP BY department;
HAVING: The HAVING clause is used to filter groups based on specific conditions. It is similar to the WHERE clause, but it is used with the GROUP BY clause.
sql
SELECT department, COUNT(*)
FROM employees
GROUP BY department
HAVING COUNT(*) > 5;
Data Types in SQL
SQL supports various data types that define the kind of data that can be stored in a table. Understanding these data types is crucial for creating and manipulating tables.
- INT: Integer numbers (e.g., 1, 2, 3)
- VARCHAR: Variable-length character strings (e.g., ‘John’, ‘Sales’)
- DATE: Dates (e.g., ‘2023-08-10’)
- FLOAT: Floating-point numbers (e.g., 3.14, 9.99)
- BOOLEAN: True or False values
Tables, Columns, and Rows
In SQL, data is stored in tables. A table consists of columns and rows. Each column represents a specific attribute, and each row represents a record in the table.
- Table: A collection of related data stored in rows and columns.
- Column: A vertical entity in a table that contains data of the same type.
- Row: A horizontal entity in a table that represents a single record.
Primary Keys, Foreign Keys, and Indexes
Primary Key: A primary key is a unique identifier for each record in a table. It ensures that no two records have the same value in the primary key column.
sql
CREATE TABLE employees (
employee_id INT PRIMARY KEY,
first_name VARCHAR(50),
last_name VARCHAR(50)
);
Foreign Key: A foreign key is a column or a set of columns in one table that refers to the primary key in another table. It establishes a relationship between the two tables.
sql
CREATE TABLE orders (
order_id INT PRIMARY KEY,
employee_id INT,
FOREIGN KEY (employee_id) REFERENCES employees(employee_id)
);
Indexes: Indexes are used to improve the performance of queries by allowing the database to find and retrieve specific rows faster. Indexes can be created on one or more columns.
sql
CREATE INDEX idx_employee_last_name
ON employees(last_name);
Next, we will look at common SQL coding interview questions. This will help you see how these fundamentals apply in real scenarios.
SQL Coding Interview Questions and Answers
Understanding SQL fundamentals is essential, but knowing how to apply them in real-world scenarios is what will set you apart in an interview. This section will cover common SQL interview questions and their solutions, helping you to be well-prepared.
Core SQL Concepts
Joins (Inner, Outer, Left, Right, Full)
Joins are used to combine rows from two or more tables based on related columns. There are several types of joins, each serving a different purpose.
Inner Join: Returns only the rows that have matching values in both tables.
sql
SELECT employees.first_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
Left Join (or Left Outer Join): Returns all rows from the left table and the matching rows from the right table. If there is no match, NULL is returned for columns from the right table.
sql
SELECT employees.first_name, departments.department_name
FROM employees
LEFT JOIN departments ON employees.department_id = departments.department_id;
Right Join (or Right Outer Join): Returns all rows from the right table and the matching rows from the left table. If there is no match, NULL is returned for columns from the left table.
sql
SELECT employees.first_name, departments.department_name
FROM employees
RIGHT JOIN departments ON employees.department_id = departments.department_id;
Full Join (or Full Outer Join): Returns all rows when there is a match in either the left or right table. If there is no match, NULL is returned for columns where there is no match.
sql
SELECT employees.first_name, departments.department_name
FROM employees
FULL OUTER JOIN departments ON employees.department_id = departments.department_id;
Subqueries
A subquery is a query nested inside another query. Subqueries are often used to perform calculations that are dependent on the results of another query.
Example: Find employees who earn more than the average salary in their department.
sql
SELECT first_name, last_name
FROM employees
WHERE salary > (SELECT AVG(salary) FROM employees);
Aggregate Functions (COUNT, SUM, AVG, MIN, MAX)
Aggregate functions perform calculations on a set of values and return a single value. They are commonly used with the GROUP BY clause.
COUNT: Returns the number of rows that match the specified condition.
sql
SELECT COUNT(*)
FROM employees;
SUM: Returns the total sum of a numeric column.
sql
SELECT SUM(salary)
FROM employees;
AVG: Returns the average value of a numeric column.
sql
SELECT AVG(salary)
FROM employees;
MIN: Returns the smallest value in a column.
sql
SELECT MIN(salary)
FROM employees;
MAX: Returns the largest value in a column.
sql
SELECT MAX(salary)
FROM employees;
Group By and Having Clauses
The GROUP BY clause groups rows that have the same values in specified columns. It is often used with aggregate functions to produce summary reports.
Example: Count the number of employees in each department.
sql
SELECT department_id, COUNT(*)
FROM employees
GROUP BY department_id;
The HAVING clause is used to filter groups based on specific conditions, similar to the WHERE clause but applied after grouping.
Example: Find departments with more than 5 employees.
sql
SELECT department_id, COUNT(*)
FROM employees
GROUP BY department_id
HAVING COUNT(*) > 5;
Case Statements and Functions
CASE statements allow you to perform conditional logic in SQL. It works like an if-then-else statement.
Example: Assign a performance category based on salary.
sql
SELECT first_name, last_name,
CASE
WHEN salary > 100000 THEN ‘High’
WHEN salary BETWEEN 50000 AND 100000 THEN ‘Medium’
ELSE ‘Low’
END AS performance_category
FROM employees;
Window Functions
Window functions perform calculations across a set of table rows that are related to the current row. They are often used for running totals, ranking, and moving averages.
Example: Rank employees based on their salary within their department.
sql
SELECT first_name, last_name, department_id, salary,
RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) AS salary_rank
FROM employees;
In this example, the RANK() function ranks employees within each department based on their salary. The PARTITION BY clause divides the result set into partitions (in this case, by department), and the ORDER BY clause sorts the rows within each partition.
Advanced SQL Topics
Understanding core SQL concepts is crucial, but SQL interviews often test your knowledge of more advanced topics as well. Mastering these advanced topics can help you stand out in an SQL coding interview.
Common Table Expressions (CTEs)
A Common Table Expression (CTE) is a temporary result set that you can reference within a SELECT, INSERT, UPDATE, or DELETE statement. CTEs improve the readability and maintainability of complex queries.
Example: Use a CTE to find employees who have a salary above the department average.
sql
WITH DepartmentAvg AS (
SELECT department_id, AVG(salary) AS avg_salary
FROM employees
GROUP BY department_id
)
SELECT e.first_name, e.last_name, e.salary
FROM employees e
JOIN DepartmentAvg d ON e.department_id = d.department_id
WHERE e.salary > d.avg_salary;
In this example, the WITH clause creates a CTE named DepartmentAvg that calculates the average salary for each department. The main query then uses this CTE to find employees who earn more than the average salary in their department.
Views
A View is a virtual table based on the result set of an SQL query. Views are useful for simplifying complex queries, enforcing security, and ensuring consistency in data retrieval.
Example: Create a view to simplify access to employees’ basic information.
sql
CREATE VIEW EmployeeInfo AS
SELECT first_name, last_name, department_id, salary
FROM employees;
You can now retrieve data from the EmployeeInfo view just as you would from a regular table.
sql
SELECT * FROM EmployeeInfo WHERE department_id = 3;
Stored Procedures and Functions
Stored procedures are precompiled collections of SQL statements that can be executed as a single unit. Functions are similar to stored procedures, but they return a single value and can be used within SQL statements.
Example: Create a stored procedure to increase the salary of employees in a specific department by a certain percentage.
sql
CREATE PROCEDURE IncreaseSalary(@DeptID INT, @Percentage FLOAT)
AS
BEGIN
UPDATE employees
SET salary = salary + (salary * @Percentage / 100)
WHERE department_id = @DeptID;
END;
You can execute this stored procedure with specific parameters:
sql
EXEC IncreaseSalary 3, 10;
Example: Create a function to calculate the annual salary of an employee.
sql
CREATE FUNCTION AnnualSalary(@EmpID INT)
RETURNS FLOAT
AS
BEGIN
DECLARE @AnnualSalary FLOAT;
SELECT @AnnualSalary = salary * 12
FROM employees
WHERE employee_id = @EmpID;
RETURN @AnnualSalary;
END;
You can use this function in a query to retrieve the annual salary of an employee:
sql
SELECT dbo.AnnualSalary(101);
Indexing and Performance Optimization
Indexes are crucial for optimising the performance of SQL queries, especially as databases grow in size. An index allows the database to find data faster, reducing the amount of time it takes to execute queries.
Example: Create an index on the last_name column of the employees table.
sql
CREATE INDEX idx_last_name ON employees(last_name);
However, while indexes can significantly speed up data retrieval, they can also slow down data modification operations (INSERT, UPDATE, DELETE) because the indexes need to be updated. Therefore, it’s important to use indexes wisely.
- Query Optimization: Besides indexing, there are other techniques to optimise SQL queries, such as:
- *Avoiding SELECT : Specify only the columns you need.
- Using WHERE Clauses: Filter data as early as possible in the query.
- Minimising Subqueries: Where possible, replace subqueries with joins or CTEs.
Database Normalisation
Normalisation is the process of organising data in a database to reduce redundancy and improve data integrity. The goal is to divide a database into two or more tables and define relationships between them.
First Normal Form (1NF): Ensures that the table has a primary key, and each column contains atomic values (no repeating groups or arrays).
Second Normal Form (2NF): Ensures that all non-key attributes are fully functional and dependent on the primary key.
Third Normal Form (3NF): Ensures that all non-key attributes are not dependent on any other non-key attributes.
Normalisation helps in designing efficient and scalable databases, but over-normalization can lead to complex queries and reduced performance. Therefore, it’s essential to find the right balance based on the specific requirements of your application.
SQL for Data Analysis and Business Intelligence
SQL is not just about managing databases; it’s also a powerful tool for data analysis and business intelligence. With SQL, you can perform complex data manipulations, generate reports, and derive insights that drive business decisions.
Data Aggregation: SQL’s aggregate functions (SUM, AVG, COUNT, etc.) are commonly used in data analysis to summarise data.
Reporting: SQL queries can be used to generate reports by aggregating data, applying filters, and formatting the output.
Data Mining: SQL can be used to extract patterns and trends from large datasets, making it a valuable tool for business intelligence.
Example: Find the total revenue generated by each product category.
sql
SELECT category_name, SUM(revenue) AS total_revenue
FROM sales
GROUP BY category_name;
This query aggregates the sales data by product category and calculates the total revenue for each category.
After this, we will provide practice questions to help you refine your skills. Practising will boost your confidence and readiness.
Practice SQL Coding Interview Questions
To succeed in an SQL coding interview, it’s important to practise a variety of SQL questions that cover different levels of difficulty and topics. Below are some practice questions categorised by difficulty level, along with detailed explanations and solutions.
Beginner Level
Question 1: Write an SQL query to find the names of all employees who work in the ‘Sales’ department.
sql
SELECT first_name, last_name
FROM employees
WHERE department = ‘Sales’;
Explanation: This query selects the first_name and last_name columns from the employees table and filters the results to include only those employees who work in the ‘Sales’ department.
Question 2: Write an SQL query to find the total number of orders placed by each customer.
sql
SELECT customer_id, COUNT(*) AS total_orders
FROM orders
GROUP BY customer_id;
Explanation: This query counts the number of orders for each customer by grouping the results by customer_id.
Intermediate Level
Question 1: Write an SQL query to find the second highest salary from the employees table.
sql
SELECT MAX(salary) AS second_highest_salary
FROM employees
WHERE salary < (SELECT MAX(salary) FROM employees);
Explanation: This query finds the maximum salary from the employees table where the salary is less than the highest salary, effectively returning the second highest salary.
Question 2: Write an SQL query to retrieve the names of employees who have more than one manager.
sql
SELECT first_name, last_name
FROM employees
WHERE employee_id IN (
SELECT employee_id
FROM employee_managers
GROUP BY employee_id
HAVING COUNT(manager_id) > 1
);
Explanation: This query uses a subquery to find employees who have more than one manager by grouping the results by employee_id and filtering with the HAVING clause.
Advanced Level
Question 1: Write an SQL query to find the top 5 employees with the highest salaries in each department.
sql
SELECT department_id, first_name, last_name, salary
FROM (
SELECT department_id, first_name, last_name, salary,
RANK() OVER (PARTITION BY department_id ORDER BY salary DESC) AS salary_rank
FROM employees
) AS ranked_employees
WHERE salary_rank <= 5;
Explanation: This query ranks employees within each department based on their salary using the RANK() function and then filters the results to include only the top 5 employees in each department.
Question 2: Write an SQL query to find all orders where the total order value exceeds the average order value for that customer.
sql
SELECT order_id, customer_id, total_order_value
FROM orders o
WHERE total_order_value > (
SELECT AVG(total_order_value)
FROM orders
WHERE customer_id = o.customer_id
);
Explanation: This query uses a subquery to calculate the average order value for each customer and then filters the orders to include only those where the total order value exceeds this average.
Once you finish practising, we will share valuable tips and strategies for your SQL interviews. These insights will help you perform your best.
SQL Interview Tips and Strategies
Preparing for an SQL coding interview requires more than just practising questions. Here are some strategies to help you perform well in your interview:
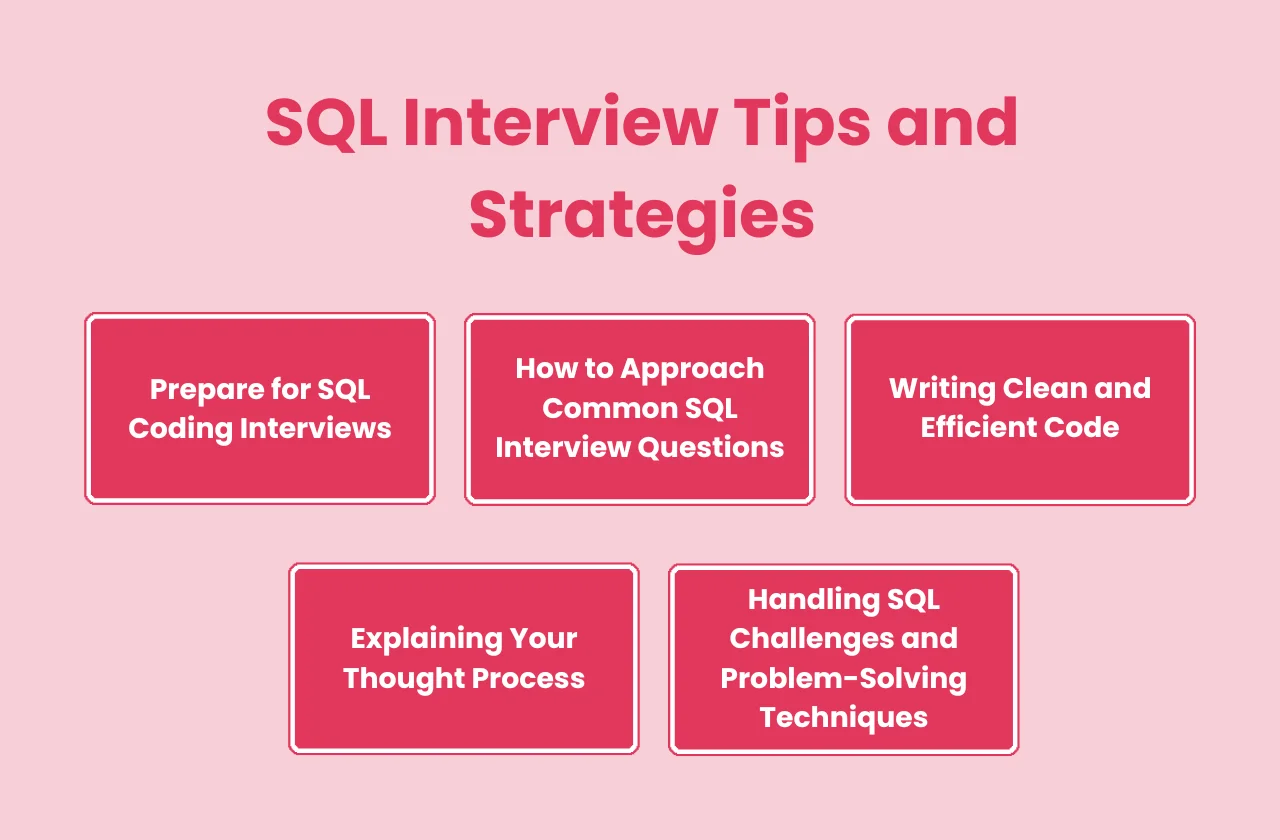
1) Prepare for SQL Coding Interviews
To excel in SQL coding interviews, consistent practice is key. iScalePro offers a wide range of SQL coding challenges that mimic the types of questions you might encounter in a real interview.
Regular practice on iScalePro platforms helps you familiarise yourself with different types of SQL problems, improve your problem-solving speed, and build confidence.
2) How to Approach Common SQL Interview Questions
When faced with a question in an SQL interview, follow these steps to ensure a structured and effective approach:
Understand the Problem Statement: Carefully read the question to understand what is being asked. Pay attention to the details, such as specific conditions, required output format, and any constraints.
Plan Your Approach: Before writing any SQL code, think through how you will solve the problem. Identify the tables involved, the relationships between them, and the SQL concepts (e.g., joins, subqueries) you need to apply.
Write the Query Step-by-Step: Start with a basic query and gradually build on it. For example, first, retrieve the necessary columns, then add conditions, and finally, apply any required grouping or sorting.
Test and Validate: If you have access to a database, test your query with different inputs to ensure it works as expected. Make sure to check for edge cases, such as null values or empty results.
Optimise If Necessary: Once you have a working solution, consider whether it can be optimised for performance. For example, you might add indexes, simplify joins, or eliminate unnecessary subqueries.
3) Writing Clean and Efficient Code
In an SQL interview, it’s important to write code that is both correct and efficient. Here are some tips for writing clean SQL code:
Use Meaningful Aliases: When working with multiple tables or columns, use aliases to make your code more readable. For example, instead of SELECT e.first_name, use SELECT emp.first_name AS employee_name.
Avoid SELECT *: Instead of using SELECT *, specify only the columns you need. This improves performance and makes your code more transparent.
Indent and Format Your Code: Proper indentation and formatting make your SQL code easier to read and understand, especially when dealing with complex queries.
Comment Your Code: If your query is complex, add comments to explain your logic. This can help the interviewer understand your thought process.
Prioritise Performance: Always consider the performance of your queries, especially when working with large datasets. Avoid full table scans if possible, and use indexes or optimised joins.
4) Explaining Your Thought Process
During an SQL interview, you’ll likely be asked to explain your thought process as you solve the problem. This is an opportunity to demonstrate your understanding of SQL concepts and your approach to problem-solving. Here’s how to effectively explain your thought process:
Start with the Problem: Begin by summarising the problem statement in your own words. This shows that you understand what is being asked.
Describe Your Approach: Explain the steps you plan to take to solve the problem. For example, if you’re using a join, explain why it’s necessary and how it helps achieve the desired result.
Discuss Alternatives: If there are multiple ways to solve the problem, mention the alternatives and why you chose the approach you did.
Highlight Key Decisions: Point out any important decisions you made while writing the query, such as using a specific function or optimising a particular part of the query.
Be Honest About Uncertainties: If you’re unsure about a part of the query, it’s okay to mention it. This shows that you’re aware of potential issues and are thinking critically about your solution.
5) Handling SQL Challenges and Problem-Solving Techniques
SQL interviews often include challenges that test your problem-solving abilities. Here are some techniques to handle these challenges effectively:
Break Down the Problem: If the problem seems complex, break it down into smaller, manageable parts. Solve each part individually, then combine them to form the final solution.
Use Visualisation: If possible, draw diagrams or use visual aids to map out the relationships between tables or the flow of data. This can help you better understand the problem.
Leverage SQL Functions: SQL offers a variety of built-in functions that can simplify complex problems. Familiarise yourself with these functions and know when to use them.
Think About Edge Cases: Consider edge cases that might cause your query to fail or return incorrect results. For example, think about how your query handles null values, duplicates, or unexpected inputs.
Practice Under Time Constraints: In a real interview, you’ll need to solve problems within a limited time. Practice solving SQL problems with a timer to simulate interview conditions and improve your time management skills.
Conclusion
SQL is a vital skill in today’s data-driven world, especially for job seekers preparing for coding interviews. Understanding SQL fundamentals, mastering core concepts, and tackling advanced topics are essential for acing SQL interviews. By practising a variety of SQL coding questions and following effective interview strategies, you can improve your problem-solving skills and increase your chances of success.
This article has provided a comprehensive guide to SQL interview preparation, covering everything from basic SQL commands to advanced topics like CTEs, views, and indexing. Additionally, the practice questions and interview tips offered here will help you build confidence and perform well in your SQL coding interviews.
As you prepare for your SQL interview, remember to stay focused, practice regularly, and approach each problem with a clear and structured mindset. With dedication and the right preparation, you’ll be well on your way to landing your dream job in the field of data and analytics.