Landing a job as a software developer in today’s competitive market requires more than just coding skills. You need to demonstrate a deep understanding of popular frameworks and technologies, and ASP.NET Core is undoubtedly a frontrunner in the world of web development.
We understand that preparing for technical interviews can be daunting. It takes time and effort to grasp complex concepts and articulate them clearly and concisely. The sheer volume of information can be overwhelming, and knowing what to focus on can be challenging.
This article serves as your comprehensive guide to ASP.NET Core interview preparation. We’ve meticulously curated the most important topics, explained them in simple terms, and structured the content for optimal learning and retention. Whether you’re a beginner or have some experience with ASP.NET Core, this guide will equip you with the knowledge and confidence to ace your next interview.
Basic ASP.NET Core Questions
In this section, you will find simple questions to assess a candidate’s understanding of the basics of ASP.NET Core. These questions will help identify candidates with a strong foundation in the framework.
1) What is ASP.NET Core?
ASP.NET Core is an open-source, cross-platform framework developed by Microsoft for building modern, cloud-enabled web applications. It represents a significant evolution from the traditional ASP.NET framework, offering enhanced performance, modularity, and flexibility.
Key Features:
- High performance: ASP.NET Core is renowned for its exceptional performance. Independent benchmarks consistently place it among the fastest web frameworks available, enabling you to build applications that respond quickly and efficiently to user requests.
- Flexible deployment: One of the key strengths of ASP.NET Core is its cross-platform nature. You can deploy your applications on various operating systems, including Windows, Linux, and macOS, providing greater flexibility and cost-effectiveness in choosing your hosting environment.
- Modular: ASP.NET Core embraces a modular design philosophy. You include only the NuGet packages and components that your application requires, resulting in smaller deployment sizes and improved performance.
- Built-in dependency injection: Dependency injection (DI) is a crucial design pattern that promotes loose coupling and testability in your code. ASP.NET Core provides robust built-in support for DI, simplifying development and making your code easier to maintain.
Cross-platform capabilities:
The cross-platform capabilities of ASP.NET Core stem from its foundation on .NET Core, a cross-platform runtime environment. This allows you to develop and deploy applications on any operating system, breaking free from the limitations of the older ASP.NET framework, which was tied to the Windows ecosystem.
2) How does ASP.NET Core differ from ASP.NET?
ASP.NET and ASP.NET Core represent two distinct generations of web development frameworks from Microsoft. While they share some similarities, there are key differences that set them apart.
Differences in framework structure:
- Monolithic vs. Modular: ASP.NET, the older framework, has a monolithic architecture where all components are tightly coupled. In contrast, ASP.NET Core adopts a modular approach, allowing you to pick and choose the components you need, resulting in leaner and more efficient applications.
- Performance: While ASP.NET offers good performance, ASP.NET Core significantly raises the bar with its optimized architecture and efficient request processing pipeline. This translates to faster response times and improved scalability for your applications.
- Cross-platform support: ASP.NET is limited to the Windows platform, while ASP.NET Core is truly cross-platform, running seamlessly on Windows, Linux, and macOS. This opens up new possibilities for deployment and hosting, allowing you to leverage the benefits of different operating systems and cloud environments.
- Dependency Injection: ASP.NET Core has built-in support for dependency injection, a powerful design pattern that promotes code maintainability and testability. While DI can be implemented in ASP.NET, it requires third-party libraries and is not as seamlessly integrated as in ASP.NET Core.
Modular design in ASP.NET Core:
The modularity of ASP.NET Core is facilitated by its reliance on NuGet packages. Instead of including a large, monolithic framework, you add individual packages for specific functionalities, such as MVC, Web API, Entity Framework Core, and more. This granular control over dependencies ensures that your application only includes the necessary components, reducing its footprint and improving performance.
3) What is the use of the Startup.cs file in ASP.NET Core?
The Startup.cs file plays a pivotal role in ASP.NET Core applications. It serves as the entry point for configuring your application’s services and request pipeline.
Startup configuration process:
When your ASP.NET Core application starts, the runtime looks for the Startup class within the Startup.cs file. This class contains methods that define how your application should be initialized and configured.
Role of ConfigureServices() and Configure() methods:
- ConfigureServices(IServiceCollection services): This method is responsible for registering services with the built-in dependency injection container. You add services like database contexts, logging providers, authentication handlers, and other components that your application depends on. These services can then be injected into controllers, middleware, and other parts of your application.
- Configure(IApplicationBuilder app, IWebHostEnvironment env): This method defines the application’s request processing pipeline. You add middleware components to the pipeline, specifying the order in which they should process incoming requests. This includes middleware for handling static files, authentication, routing, and more. The IWebHostEnvironment parameter provides information about the current hosting environment, allowing you to configure your application differently for development, staging, and production.
4) What is middleware in ASP.NET Core?
Middleware forms the backbone of request processing in ASP.NET Core. They are components that handle incoming HTTP requests and outgoing responses, forming a pipeline through which requests flow.
Definition and role in the request pipeline:
Each middleware component in the pipeline has the opportunity to:
- Process the request: A middleware component can inspect and modify the incoming request, such as adding headers, logging information, or performing authentication.
- Generate a response: If a middleware component is designed to handle a specific type of request, it can generate the response directly, short-circuiting the rest of the pipeline.
- Pass the request to the next middleware: If a middleware component doesn’t need to handle the request itself, it passes it along to the next component in the pipeline.
Examples of common middleware:
- Authentication Middleware: This middleware is responsible for authenticating users, ensuring that only authorized users can access protected resources.
- Static Files Middleware: This middleware serves static files, such as CSS stylesheets, JavaScript files, and images, directly to the client.
- Routing Middleware: This middleware matches incoming requests to specific controllers and actions based on the defined routes.
- Session Middleware: This middleware enables session management, allowing you to store user-specific data across multiple requests.
- CORS Middleware: This middleware handles Cross-Origin Resource Sharing (CORS), allowing your application to accept requests from different origins.
- Exception Handling Middleware: This middleware catches unhandled exceptions and provides a mechanism for logging errors and displaying custom error pages.
5) Explain the concept of dependency injection in ASP.NET Core.
Dependency injection (DI) is a fundamental design pattern in ASP.NET Core. It promotes loose coupling between classes by providing a mechanism for injecting dependencies into objects rather than having them create their own.
Built-in DI support:
ASP.NET Core has a robust built-in DI container that manages the creation and lifecycle of services. You register your services with the container in the ConfigureServices() method of the Startup class, and the container takes care of resolving dependencies and providing instances of services to the classes that need them.
Role of services and containers:
Services: A service is any object that your application depends on to perform its functions. This can include database contexts, logging providers, email senders, and any other component that provides a specific functionality.
Containers: The DI container acts as a central registry for your services. It is responsible for creating instances of services and resolving their dependencies. When a class needs a particular service, it requests it from the container, and the container provides the appropriate instance.
Benefits of Dependency Injection:
- Loose Coupling: DI reduces the coupling between classes, making your code more modular and easier to maintain. Changes to one class are less likely to affect other classes that depend on it.
- Testability: DI makes it easier to write unit tests for your code. You can easily mock or stub out dependencies, allowing you to test individual components in isolation.
- Maintainability: DI promotes code reuse and makes it easier to refactor your code. You can easily swap out implementations of services without affecting the classes that depend on them.
6) What is Kestrel, and why is it important?
Kestrel is the default web server for ASP.NET Core applications. It’s a cross-platform, high-performance web server built on libuv, an asynchronous I/O library.
Introduction to Kestrel web server:
Kestrel is designed to be lightweight and efficient, capable of handling a large number of concurrent requests. It’s a key component of ASP.NET Core’s ability to achieve excellent performance.
Advantages in performance and cross-platform support:
Performance: Kestrel’s architecture and use of asynchronous I/O make it highly performant. It can handle many requests per second with minimal resource consumption.
Cross-platform: Kestrel runs on Windows, Linux, and macOS, making it ideal for deploying ASP.NET Core applications to any platform. This is a significant advantage over traditional web servers like IIS, which are limited to Windows.
When to use a reverse proxy with Kestrel:
While Kestrel is a capable web server, it’s often used in conjunction with a reverse proxy like IIS, Nginx, or Apache in production environments. A reverse proxy provides additional features such as:
- Load balancing: Distributing incoming traffic across multiple instances of your application.
- SSL termination: Handling SSL encryption and decryption.
- Static file serving: Efficiently serving static files like images and CSS.
- Security: Protecting your application from attacks.
7) How do you create a new ASP.NET Core project?
You have two primary options for creating new ASP.NET Core projects: the .NET CLI and Visual Studio.
Project setup using CLI and Visual Studio:
.NET CLI: The .NET CLI is a cross-platform command-line interface for working with .NET projects. To create a new ASP.NET Core web application, open your terminal or command prompt and run the command dotnet new web. You can specify different templates for creating different types of ASP.NET Core applications, such as Web API (dotnet new webapi), Razor Pages (dotnet new razor), and MVC (dotnet new mvc).
Visual Studio: Visual Studio provides a user-friendly graphical interface for creating and managing ASP.NET Core projects. You can choose from a variety of project templates, configure your project settings, and start developing your application with the help of Visual Studio’s powerful code editor and debugging tools.
Command syntax (dotnet new, dotnet run):
dotnet new <template>: This command creates a new project based on the specified template. For example, dotnet new webapi creates a new ASP.NET Core Web API project.
dotnet run: This command builds and runs your ASP.NET Core application. It launches the Kestrel web server and makes your application accessible in your web browser.
8) Explain the ASP.NET Core request processing pipeline.
The request processing pipeline is a core concept in ASP.NET Core. It defines how incoming requests are handled and how responses are generated. The pipeline is composed of a series of middleware components that process requests sequentially.
Flow of requests through middleware:
- A request arrives at the ASP.NET Core application.
- The request enters the first middleware component in the pipeline.
- Each middleware component has the opportunity to inspect and modify the request or generate a response.
- If a middleware component doesn’t generate a response, it passes the request to the next middleware component in the pipeline.
- This process continues until the request reaches the end of the pipeline or a middleware component generates a response.
- The response travels back through the pipeline, allowing each middleware component to modify it before it is sent back to the client.
Importance of Use and Run methods:
- app.Use(middleware): This method adds a middleware component to the request pipeline. The middleware can choose to pass the request to the next middleware in the pipeline using the next delegate.
- app.Run(middleware): This method adds a terminal middleware component to the pipeline. This component is always the last one to be executed, and it typically generates the final response. It does not call the next delegate, effectively ending the pipeline.
Example of middleware order:
C#
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// … other middleware …
app.UseHttpsRedirection(); // Redirect HTTP requests to HTTPS
app.UseStaticFiles(); // Serve static files
app.UseRouting(); // Match requests to routes
app.UseAuthentication(); // Authenticate users
app.UseAuthorization(); // Authorize users
app.UseEndpoints(endpoints =>
{
endpoints.MapControllers(); // Map controllers for API requests
endpoints.MapRazorPages(); // Map Razor Pages for page-based requests
});
// … other middleware …
}
9) How does routing work in ASP.NET Core?
Routing is the mechanism that maps incoming URLs to specific controllers and actions within your ASP.NET Core application. It determines how your application responds to different requests.
URL routing and attribute-based routing:
Conventional Routing: You define routing rules in the Startup.cs file, specifying URL patterns and mapping them to controllers and actions. This approach provides a centralized way to manage your application’s routes.
C#
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: “default”,
pattern: “{controller=Home}/{action=Index}/{id?}”);
});
Attribute-based Routing: You use attributes like [HttpGet], [HttpPost], [Route], and [ApiController] directly on your controllers and actions to define routes. This approach provides a more decentralized and declarative way to specify routing information.
C#
[ApiController]
[Route(“api/[controller]”)]
public class ProductsController : ControllerBase
{
[HttpGet(“{id}”)]
public IActionResult GetProduct(int id) { … }
}
Configuring routes in Startup.cs:
You configure routing in the Configure() method of the Startup class using the app.UseRouting() and app.UseEndpoints() methods. UseRouting() adds the routing middleware to the pipeline, and UseEndpoints() defines the endpoints and their associated routes.
10) What are the main differences between MVC and Razor Pages in ASP.NET Core?
ASP.NET Core offers two primary approaches for building web applications: MVC (Model-View-Controller) and Razor Pages. While both can be used to create web applications, they differ in their structure and philosophy.
Key concepts of MVC architecture:
- Model: Represents the data and business logic of your application.
- View: Displays the data to the user and provides a user interface.
- Controller: Handles user input, interacts with the model, and selects the appropriate view to render.
MVC promotes separation of concerns, making your code more organized and maintainable.
Overview of Razor Pages as a page-focused model:
Razor Pages provide a simpler, page-centric approach to building web applications. Each Razor Page consists of a .cshtml file (the view) and an optional .cshtml.cs file (the PageModel). The PageModel handles user input, interacts with the model, and provides data to the view.
Key differences:
Feature | MVC | Razor Pages |
Structure | Controllers and Views | Pages and PageModels |
Focus | Separation of concerns | Page-centric development |
Complexity | Can be more complex for simple applications | Simpler for page-based applications |
Organization | Controllers organized by functionality | Pages organized by feature or flow |
Routing | Typically uses conventional routing | Uses a file-based routing convention |
Choosing between MVC and Razor Pages:
- MVC: Suitable for complex applications with many controllers and actions, where separation of concerns is paramount.
- Razor Pages: Ideal for simpler, page-focused applications where the focus is on individual pages and their associated logic.
Once you have evaluated the basics, it’s time to move on to intermediate-level questions to test their practical knowledge.
Intermediate ASP.NET Core Questions
This section includes questions that focus on real-world usage and practical implementation of ASP.NET Core. These will help you assess a candidate’s ability to apply their skills in a work environment.
11) What are the different hosting models available in ASP.NET Core?
ASP.NET Core supports two main hosting models: in-process and out-of-process.
In-process hosting vs. out-of-process hosting:
- In-process hosting: In this model, your ASP.NET Core application runs within the same process as the web server (e.g., IIS). This provides improved performance due to reduced inter-process communication overhead.
- Out-of-process hosting: In this model, your ASP.NET Core application runs in a separate process from the web server. This offers better isolation and fault tolerance. If your application crashes, it won’t bring down the web server.
Use of IIS, Kestrel, and Nginx:
- IIS: Internet Information Services (IIS) is Microsoft’s web server for Windows. When hosting ASP.NET Core applications in-process, IIS acts as the host and directly handles requests. For out-of-process hosting, IIS acts as a reverse proxy, forwarding requests to Kestrel.
- Kestrel: Kestrel is the default, cross-platform web server for ASP.NET Core. It’s designed for performance and efficiency. In out-of-process hosting, Kestrel is the primary web server that receives requests and passes them to your application.
- Nginx: Nginx is another popular open-source web server that can be used as a reverse proxy for Kestrel, especially in Linux environments. It offers features similar to IIS, including load balancing, SSL termination, and static file serving.
12) How does the environment configuration system work in ASP.NET Core?
ASP.NET Core provides a flexible configuration system that allows you to load different settings for different environments, such as development, staging, and production.
Use of environment variables (Development, Staging, Production):
The ASPNETCORE_ENVIRONMENT environment variable plays a key role in determining the current environment. You can set this variable to “Development”, “Staging”, or “Production” to indicate the environment in which your application is running.
appsettings.json and environment-specific settings:
The primary configuration file in ASP.NET Core is appsettings.json. This file stores default settings for your application. You can also create environment-specific versions of this file, such as appsettings.Development.json and appsettings.Production.json. ASP.NET Core automatically loads the appropriate configuration file based on the value of the ASPNETCORE_ENVIRONMENT variable.
Configuration providers:
ASP.NET Core supports multiple configuration providers, allowing you to load settings from various sources, including:
- JSON files
- XML files
- Environment variables
- Command-line arguments
- Azure Key Vault
- In-memory collections
This flexibility allows you to tailor your configuration to different deployment scenarios and environments.
13) What is the purpose of the IApplicationBuilder interface?
The IApplicationBuilder interface is central to configuring the request processing pipeline in ASP.NET Core. It provides methods for adding middleware components to the pipeline and defining how requests are handled.
Configuring middleware in the application pipeline:
You use the IApplicationBuilder instance in the Configure() method of your Startup class to build the request pipeline. You add middleware components using methods like Use(), Run(), and Map().
Role in adding middleware components:
The IApplicationBuilder ensures that middleware components are added to the pipeline in the correct order. Each middleware component has the opportunity to process the request and either pass it to the next middleware or generate a response.
Key methods of IApplicationBuilder:
Use(Func<HttpContext, Func<Task>, Task>): Adds a middleware delegate to the pipeline.
Run(RequestDelegate): Adds a terminal middleware delegate to the pipeline.
Map(PathString, Action<IApplicationBuilder>): Branches the request pipeline based on the specified path.
UseWhen(Func<HttpContext, bool>, Action<IApplicationBuilder>): Conditionally adds middleware to the pipeline based on a predicate.
14) How do you handle exceptions and errors in ASP.NET Core?
Exception handling is crucial for building robust and reliable ASP.NET Core applications. ASP.NET Core provides several mechanisms for handling exceptions and errors gracefully.
Exception handling middleware:
The UseExceptionHandler() middleware allows you to catch unhandled exceptions that occur in your application. You can configure this middleware to:
Log the exception details.
Redirect the user to a custom error page.
Execute custom error handling logic.
Custom error pages:
You can create custom error pages for different HTTP status codes (e.g., 404 Not Found, 500 Internal Server Error). This allows you to provide user-friendly error messages and guidance to users when something goes wrong.
Logging:
ASP.NET Core has built-in support for logging. You can configure logging to write to various destinations, including the console, files, and databases. Logging exceptions provides valuable information for debugging and troubleshooting your application.
Global exception filters:
Global exception filters allow you to handle exceptions globally across your application. You can implement the IExceptionFilter interface to create a custom global exception filter that catches and handles exceptions before they are propagated to the exception handling middleware.
15) How does session management work in ASP.NET Core?
Session management allows you to store user-specific data across multiple requests. This is essential for maintaining state in web applications, such as storing shopping cart items, user preferences, and login information.
Working with sessions and session storage:
ASP.NET Core provides different session storage options:
- In-memory session storage: Stores session data in the memory of the web server. This is the default option and is suitable for simple applications.
- Distributed session storage: Stores session data in a distributed cache, such as SQL Server, Redis, or Azure Cache for Redis. This is necessary for applications deployed to multiple servers or cloud environments.
Configuring session timeout and persistence:
You configure session management in the ConfigureServices() method of the Startup class using the AddSession() method. You can specify options such as:
IdleTimeout: The maximum duration of inactivity before the session expires.
Cookie.Name: The name of the cookie used to store the session ID.
Cookie.Path: The path for which the cookie is valid.
Cookie.SecurePolicy: Specifies whether the cookie should only be transmitted over HTTPS.
16) What is CORS and how do you configure it in ASP.NET Core?
CORS (Cross-Origin Resource Sharing) is a security mechanism implemented by web browsers to prevent web pages from making requests to a different domain than the one that served the web page.
Cross-Origin Resource Sharing (CORS) basics:
By default, browsers restrict cross-origin requests to prevent malicious websites from accessing sensitive data on another domain. CORS provides a way to relax these restrictions and allow controlled cross-origin access.
Enabling and configuring CORS policy:
You enable and configure CORS in ASP.NET Core in the ConfigureServices() method of the Startup class using the AddCors() method. You can define specific CORS policies to control which origins, methods, and headers are allowed.
Example CORS policy:
C#
services.AddCors(options =>
{
options.AddPolicy(“MyCorsPolicy”,
builder =>
{
builder.WithOrigins(“https://www.example.com”)
.AllowAnyMethod()
.AllowAnyHeader();
});
});
Applying CORS policy:
You apply a CORS policy to a controller or action using the [EnableCors] attribute.
C#
[EnableCors(“MyCorsPolicy”)]
[ApiController]
[Route(“api/[controller]”)]
public class ProductsController : ControllerBase
{
// …
}
17) What are filters in ASP.NET Core?
Filters are attributes that you can apply to controllers or actions to run code before, after, or around the execution of an action method. They provide a way to implement cross-cutting concerns, such as logging, authorization, and exception handling.
Types of filters (authorization, action, result, resource, exception):
- Authorization filters: Control access to action methods. They run before other filters and can short-circuit the request if authorization fails.
- Action filters: Execute code before and after an action method is executed. They can be used for tasks like logging, caching, and input validation.
- Result filters: Execute code before and after an action result is executed. They can be used for tasks like modifying the response or logging the result.
- Resource filters: Execute code before and after the execution of an action method, including model binding and action result execution. They are a combination of action filters and result filters.
- Exception filters: Handle exceptions that occur during the execution of an action method. They can be used to log exceptions or provide custom error responses.
Use cases for custom filters:
You can create custom filters to encapsulate specific logic and apply it to multiple controllers or actions. This promotes code reuse and maintainability.
18) Explain Model Binding and Model Validation in ASP.NET Core.
Model binding and model validation are essential aspects of handling user input in ASP.NET Core applications.
Binding form data to models:
Model binding is the process of mapping data from an HTTP request to the parameters of an action method. ASP.NET Core automatically binds data from various sources, including:
- Form data
- Route values
- Query string parameters
- HTTP headers
This simplifies the process of retrieving user input and working with it in your code.
Server-side validation using data annotations:
Model validation ensures that the data submitted by the user meets the requirements of your application. You can use data annotations to define validation rules for your model properties. Common data annotations include:
[Required]
[StringLength]
[Range]
[EmailAddress]
ASP.NET Core automatically performs validation and provides error messages if the data is invalid.
19) What are Tag Helpers in ASP.NET Core?
Tag Helpers are a feature of Razor views that allow you to write server-side code in your views using HTML-like syntax. They provide a more natural and intuitive way to generate HTML and interact with server-side logic.
Purpose and usage of tag helpers:
Tag Helpers make your Razor views cleaner and easier to read by encapsulating server-side logic within HTML tags. They can be used for tasks like:
- Generating form elements
- Creating links
- Adding validation attributes
- Loading scripts and stylesheets
Common built-in tag helpers (e.g., form, input, anchor, label):
ASP.NET Core provides a wide range of built-in tag helpers, including:
- asp-for: Generates a label and input element for a model property.
- asp-validation-for: Displays validation messages for a model property.
- asp-action: Creates a link to an action method.
- asp-route: Adds route values to a link.
- asp-page: Creates a link to a Razor Page.
Creating custom tag helpers:
You can also create your own custom tag helpers to encapsulate specific logic and reuse it across your application.
20) How do you work with static files in ASP.NET Core?
Static files are files that your web application serves directly to clients, such as CSS stylesheets, JavaScript files, images, and fonts.
Serving static files with UseStaticFiles():
The UseStaticFiles() middleware is used to serve static files from the wwwroot folder in your ASP.NET Core project. This middleware should be added to the request pipeline in the Configure() method of the Startup class.
Configuring default file and directory browsing:
You can configure a default file (e.g., index.html) to be served when a user requests a directory. You can also enable or disable directory browsing, which allows users to view the contents of a directory if no default file is found.
UseFileServer():
The UseFileServer() middleware combines the functionality of UseStaticFiles(), UseDefaultFiles(), and UseDirectoryBrowser(). It provides a convenient way to serve static files with optional default file handling and directory browsing.
After evaluating their intermediate knowledge, you can dive into advanced questions to check their expertise.
Advanced ASP.NET Core Questions
Advanced questions help you identify candidates with deep knowledge and problem-solving abilities. These questions focus on complex scenarios and advanced features of ASP.NET Core.
21) How does authentication and authorization work in ASP.NET Core?
Authentication and authorization are critical security aspects of web applications. ASP.NET Core provides a comprehensive framework for implementing authentication and authorization.
Difference between authentication and authorization:
- Authentication: The process of verifying the identity of a user. It involves confirming that the user is who they claim to be.
- Authorization: The process of determining what a user is allowed to do after they have been authenticated. It involves checking if the user has the necessary permissions to access a particular resource or perform a specific action.
Role of Identity framework and JWT (JSON Web Tokens):
- ASP.NET Core Identity: A membership system that provides features for managing users, roles, passwords, claims, and more. It simplifies the process of building user authentication and authorization into your applications.
- JWT (JSON Web Tokens): An open standard (RFC 7519) that defines a compact and self-contained way for securely transmitting information between parties as a JSON object. JWTs are commonly used for authentication and authorization in web applications and APIs.
22) How do you implement token-based authentication in ASP.NET Core?
Token-based authentication is a popular approach to authentication in modern web applications and APIs. It involves issuing tokens to users after they have been authenticated, and these tokens are then used to authorize subsequent requests.
Overview of JWT authentication:
JWT (JSON Web Token) is a common standard for implementing token-based authentication. A JWT consists of three parts:
- Header: Contains information about the token type and the hashing algorithm used.
- Payload: Contains claims, which are statements about an entity (typically, the user) and additional data.
- Signature: Ensures the integrity of the token. It is created by signing the header and payload with a secret key.
Configuring authentication middleware:
You configure JWT authentication in ASP.NET Core using the AddAuthentication() and AddJwtBearer() methods in the ConfigureServices() method of the Startup class.
Example JWT authentication configuration:
C#
services.AddAuthentication(JwtBearerDefaults.AuthenticationScheme)
.AddJwtBearer(options =>
{
options.TokenValidationParameters = new TokenValidationParameters
{
ValidateIssuer = true,
ValidateAudience = true,
ValidateLifetime = true,
ValidateIssuerSigningKey = true,
ValidIssuer = Configuration[“Jwt:Issuer”],
ValidAudience = Configuration[“Jwt:Audience”],
IssuerSigningKey = new SymmetricSecurityKey(Encoding.UTF8.GetBytes(Configuration[“Jwt:Key”]))
};
});
23) What is ASP.NET Core Identity, and how do you use it?
ASP.NET Core Identity is a powerful membership system that provides a framework for managing users, roles, and claims in your applications. It simplifies the process of adding user authentication and authorization features.
Managing users, roles, and claims:
ASP.NET Core Identity provides features for:
- User management: Creating, updating, deleting, and managing user accounts.
- Password management: Hashing and storing passwords securely, handling password resets, and enforcing password policies.
- Role management: Creating roles, assigning users to roles, and using roles for authorization.
- Claim management: Adding claims to users and using claims for authorization.
Customizing Identity for extended functionality:
You can customize ASP.NET Core Identity to meet the specific needs of your application. You can:
- Extend the user model to add custom properties.
- Customize the user interface (UI) for registration, login, and password management.
- Implement custom password hashing and validation logic.
- Integrate with external authentication providers.
24) How do you implement OAuth and OpenID Connect in ASP.NET Core?
OAuth 2.0 and OpenID Connect (OIDC) are industry-standard protocols for authorization. They allow users to grant third-party applications access to their resources without having to share their credentials.
Introduction to OAuth2 and OpenID Connect protocols:
- OAuth 2.0: An authorization framework that enables applications to obtain limited access to user accounts on an HTTP service, such as Facebook, Google, or Microsoft Account.
- OpenID Connect: An identity layer on top of OAuth 2.0 that allows clients to verify the identity of the end-user based on the authentication performed by an authorization server, as well as to obtain basic profile information about the end-user in an interoperable and REST-like manner.
Configuring third-party login providers (Google, Facebook):
ASP.NET Core provides built-in support for integrating with OAuth 2.0 and OpenID Connect providers. You can configure your application to allow users to log in using their Google, Facebook, Twitter, or other accounts.
Example Google authentication configuration:
C#
services.AddAuthentication()
.AddGoogle(options =>
{
IConfigurationSection googleAuthNSection =
Configuration.GetSection(“Authentication:Google”);
options.ClientId = googleAuthNSection[“ClientId”];
options.ClientSecret = googleAuthNSection[“ClientSecret”];
});
25) What is gRPC in ASP.NET Core?
gRPC (Google Remote Procedure Call) is a modern, high-performance framework for building distributed applications and microservices. It’s an open-source, language-agnostic framework that uses HTTP/2 for transport and Protocol Buffers (protobuf) for serialization.
Overview of gRPC communication protocol:
gRPC allows client and server applications to communicate transparently, as if they were local to each other. It supports various communication patterns, including:
- Unary RPC: A client sends a single request to the server and receives a single response.
- Server streaming RPC: A client sends a single request to the server and receives a stream of responses.
- Client streaming RPC: A client sends a stream of requests to the server and receives a single response.
- Bidirectional streaming RPC: Both the client and server send a stream of messages to each other.
Benefits over REST for high-performance microservices:
- Performance: gRPC is generally faster and more efficient than REST due to its use of binary serialization and HTTP/2.
- Efficiency: gRPC messages are smaller than JSON payloads used in REST, resulting in reduced bandwidth usage.
- Streaming: gRPC supports bidirectional streaming, which is not easily achievable with REST.
- Type safety: gRPC uses Protocol Buffers, which provide type safety and reduce the risk of errors due to data type mismatches.
26) How do you create a Web API in ASP.NET Core?
Creating a Web API in ASP.NET Core is straightforward. You can use the .NET CLI or Visual Studio to create a new Web API project.
Setting up API controllers:
API controllers in ASP.NET Core inherit from ControllerBase and use the [ApiController] attribute to indicate that they are API controllers. This attribute enables features such as automatic HTTP 400 responses for invalid model state and inference of binding sources for action parameters.
Using [HttpGet], [HttpPost], and other action attributes:
You use attributes like [HttpGet], [HttpPost], [HttpPut], and [HttpDelete] to specify the HTTP methods that your API actions respond to. You can also use the [Route] attribute to define the routes for your API actions.
Example API controller:
C#
public class ProductsController : ControllerBase
{
[HttpGet]
public IEnumerable<Product> GetProducts() { … }
[HttpGet(“{id}”)]
public Product GetProduct(int id) { … }
[HttpPost]
public IActionResult CreateProduct(Product product) { … }
[HttpPut(“{id}”)]
public IActionResult UpdateProduct(int id, Product product) { … }
[HttpDelete(“{id}”)]
public IActionResult DeleteProduct(int id) { … }
}
27) How do you secure an ASP.NET Core Web API?
Securing your Web API is crucial to protect your data and prevent unauthorized access. ASP.NET Core provides various mechanisms for securing your APIs.
Implementing authentication and authorization for APIs:
- Authentication: You can use various authentication methods, such as JWT token authentication, API keys, or OAuth 2.0, to authenticate clients that access your API.
- Authorization: You can use authorization policies to control access to different API endpoints based on user roles, claims, or other criteria.
Securing endpoints using JWT tokens:
JWT tokens are a common way to secure APIs. You can require clients to include a valid JWT token in the Authorization header of their requests. The JWT authentication middleware validates the token and extracts the user’s identity and claims.
Other security measures:
- HTTPS: Always use HTTPS to encrypt communication between clients and your API.
- Input validation: Validate all input from clients to prevent injection attacks and other security vulnerabilities.
- Rate limiting: Limit the number of requests that a client can make within a specific time period to prevent denial-of-service (DoS) attacks.
- CORS: Configure CORS policies to control which origins are allowed to access your API.
28) What are the different ways to deploy an ASP.NET Core application?
ASP.NET Core offers flexible deployment options, allowing you to deploy your applications to various platforms and environments.
Self-contained vs. framework-dependent deployment:
- Self-contained deployment (SCD): Includes the .NET Core runtime and all dependencies with your application. This creates a larger deployment package but eliminates the need for the .NET Core runtime to be installed on the target machine.
- Framework-dependent deployment (FDD): Relies on the .NET Core runtime being installed on the target machine. This creates a smaller deployment package but requires the target machine to have the correct version of the .NET Core runtime installed.
Deployment options (Azure, Docker, IIS):
- Azure: Microsoft’s cloud platform offers various services for deploying ASP.NET Core applications, including Azure App Service, Azure Kubernetes Service (AKS), Azure Virtual Machines, and Azure Container Instances.
- Docker: A containerization platform that allows you to package your application and its dependencies into a Docker image. This image can then be deployed to any environment that supports Docker.
- IIS: Internet Information Services (IIS) is Microsoft’s web server for Windows. You can deploy ASP.NET Core applications to IIS using in-process or out-of-process hosting.
29) How does health monitoring work in ASP.NET Core?
Health checks allow you to monitor the health of your ASP.NET Core application and its dependencies. They provide a way to check if your application is running correctly and if its critical components, such as databases and external services, are available.
Implementing health checks for ASP.NET Core services:
You can use the Microsoft.Extensions.Diagnostics.HealthChecks package to add health checks to your application. This package provides a framework for defining health checks and exposing them as endpoints.
Using Microsoft.Extensions.Diagnostics.HealthChecks:
You can configure health checks for various components, including:
- Databases (SQL Server, MySQL, PostgreSQL)
- External services (APIs, message queues)
- System resources (memory, disk space)
- Custom checks
Health check endpoints:
Health checks are exposed as endpoints that return a health status (healthy, degraded, or unhealthy). You can use these endpoints to monitor your application’s health from monitoring tools or load balancers.
30) How do you handle real-time communication in ASP.NET Core?
SignalR is a library that simplifies adding real-time functionality to your ASP.NET Core applications. It allows you to push data from the server to clients instantly.
Overview of SignalR for real-time applications:
SignalR provides an abstraction over various transport protocols, including WebSockets, Server-Sent Events (SSE), and long polling. It negotiates the best transport protocol based on the client and server capabilities.
Setting up SignalR hubs and clients:
- Hubs: Server-side components that handle real-time communication. They define methods that can be called by clients and can send messages to clients.
- Clients: Connect to SignalR hubs and receive real-time updates. Clients can be implemented in various technologies, including JavaScript, .NET, and Java.
Use cases for SignalR:
Chat applications
Real-time dashboards
Collaborative tools
Online games
Notifications
Once you’ve tested their expertise, it’s important to evaluate their understanding of performance and optimization techniques in ASP.NET Core.
ASP.NET Core Performance and Optimization Questions
Performance is crucial for building scalable and efficient applications. This section covers questions that focus on optimizing ASP.NET Core applications.
31) What are some strategies for improving the performance of ASP.NET Core applications?
Performance optimization is essential for building fast and efficient ASP.NET Core applications. Here are some strategies to improve performance:
- Caching: Store frequently accessed data in a cache to reduce database access and improve response times. ASP.NET Core supports various caching mechanisms, including in-memory caching, distributed caching, and response caching.
- Minimizing middleware: Use only the necessary middleware components in your request pipeline. Each middleware component adds overhead, so minimizing the number of components can improve performance.
- Optimizing database queries: Write efficient database queries and use techniques like indexing and query optimization to reduce database load.
- Using asynchronous programming: Use asynchronous programming techniques to avoid blocking threads and improve responsiveness.
- Compressing responses: Compress responses to reduce the amount of data transferred over the network.
- Optimizing images and static files: Optimize images and other static files to reduce their size and improve loading times.
- Using a Content Delivery Network (CDN): Serve static content from a CDN to reduce latency and improve performance for users in different geographical locations.
32) How do you implement caching in ASP.NET Core?
Caching is a powerful technique for improving the performance of ASP.NET Core applications. ASP.NET Core supports various caching mechanisms.
Types of caching (in-memory, distributed, output, response):
- In-memory caching: Stores data in the memory of the web server. This is the simplest caching option and is suitable for small-scale applications.
- Distributed caching: Stores data in a distributed cache, such as Redis or SQL Server. This allows you to share cached data across multiple servers and improve scalability.
- Output caching: Caches the output of an action method or Razor Page. This can significantly improve performance for pages that are accessed frequently and don’t change often.
- Response caching: Caches entire HTTP responses, including headers and content. This can improve performance for static content and APIs.
Configuring cache expiration and sliding expiration:
You can configure cache expiration policies to control how long cached data remains valid. You can use absolute expiration, which sets a fixed expiration time, or sliding expiration, which extends the expiration time each time the cached data is accessed.
33) What is response compression, and how do you enable it in ASP.NET Core?
Response compression reduces the size of HTTP responses, resulting in faster page load times and reduced bandwidth usage.
Benefits of compressing responses:
- Faster page load times: Smaller responses are transferred faster over the network.
- Reduced bandwidth usage: Conserves bandwidth and reduces costs.
- Improved user experience: Users experience faster loading times and smoother interactions.
Configuring compression middleware:
You can enable response compression in ASP.NET Core using the UseResponseCompression() middleware. This middleware compresses responses using gzip or Brotli compression algorithms.
Example response compression configuration:
C#
public void ConfigureServices(IServiceCollection services)
{
services.AddResponseCompression(options =>
{
options.Providers.Add<GzipCompressionProvider>();
options.MimeTypes = ResponseCompressionDefaults.MimeTypes.Concat(
new[] { “image/svg+xml” });
});
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
// … other middleware …
app.UseResponseCompression();
// … other middleware …
}
34) How do you handle long-running tasks in ASP.NET Core?
Long-running tasks can block the main thread and affect the responsiveness of your ASP.NET Core application. It’s important to handle these tasks asynchronously to avoid blocking and maintain a responsive user experience.
Using background services (e.g., IHostedService):
Background services are a mechanism for running tasks in the background without blocking the main thread. You can implement the IHostedService interface to create a background service that performs long-running operations, such as processing messages from a queue or performing scheduled tasks.
Queue-based task execution:
You can use a message queue, such as Azure Queue Storage or RabbitMQ, to offload long-running tasks to a separate worker process. This allows your web application to remain responsive while the worker process handles the tasks asynchronously.
35) How do you manage database connections efficiently in ASP.NET Core?
Efficient database connection management is crucial for the performance and scalability of your ASP.NET Core applications.
Connection pooling and retry policies:
- Connection pooling: A technique for reusing database connections to reduce the overhead of creating new connections for each request. ASP.NET Core automatically uses connection pooling with ADO.NET providers.
- Retry policies: Implement retry policies to handle transient errors that can occur when connecting to a database. This improves the reliability of your application and prevents unnecessary failures.
Managing DbContext lifetime in Entity Framework Core:
The DbContext in Entity Framework Core represents a session with the database. It’s important to manage the lifetime of the DbContext efficiently to avoid resource leaks and performance issues.
- Scoped lifetime: The recommended lifetime for the DbContext in web applications. A new DbContext instance is created for each HTTP request and disposed of at the end of the request.
- Transient lifetime: A new DbContext instance is created each time it is injected. This is less efficient than scoped lifetime but can be necessary in some scenarios.
- Singleton lifetime: A single DbContext instance is shared across the entire application. This is generally not recommended for web applications.
Now that performance is covered, let’s move on to questions about testing and debugging in ASP.NET Core.
ASP.NET Core Testing and Debugging Questions
Testing and debugging are essential for delivering high-quality applications. This section includes questions to help you assess a candidate’s skills in these areas.
36) How do you write unit tests for ASP.NET Core applications?
Unit testing is an essential practice for ensuring the quality and correctness of your ASP.NET Core code. It involves testing individual units of code in isolation to verify their behavior.
Role of dependency injection in testing:
Dependency injection makes it easier to write unit tests by allowing you to mock or stub out dependencies. This allows you to test your code without relying on external dependencies, such as databases or external services.
Mocking services and controllers:
You can use mocking frameworks, such as Moq or NSubstitute, to create mock objects that simulate the behavior of real dependencies. This allows you to control the behavior of dependencies and test different scenarios.
Example unit test using Moq:
C#
[Test]
public void GetProduct_ReturnsProduct_WhenProductExists()
{
// Arrange
var mockProductService = new Mock<IProductService>();
mockProductService.Setup(service => service.GetProduct(1))
.Returns(new Product { Id = 1, Name = “Test Product” });
var controller = new ProductsController(mockProductService.Object);
// Act
var result = controller.GetProduct(1);
// Assert
var okResult = Assert.IsType<OkObjectResult>(result);
var product = Assert.IsType<Product>(okResult.Value);
Assert.Equal(1, product.Id);
Assert.Equal(“Test Product”, product.Name);
}
37) What is integration testing in ASP.NET Core, and how do you implement it?
Integration testing involves testing multiple components of your application together to verify their interactions and ensure that they work correctly as a whole.
Difference between unit and integration tests:
- Unit tests: Test individual units of code in isolation.
- Integration tests: Test how different parts of your application work together.
Setting up test projects with TestServer:
ASP.NET Core provides a TestServer class that allows you to create a test instance of your web application. You can use this test server to send HTTP requests to your application and verify the responses.
Example integration test:
C#
[Test]
public async Task GetProducts_ReturnsOkResponse_WithListOfProducts()
{
// Arrange
var client = _factory.CreateClient();
// Act
var response = await client.GetAsync(“/api/products”);
// Assert
response.EnsureSuccessStatusCode();
var products = await response.Content.ReadAsAsync<List<Product>>();
Assert.NotEmpty(products);
}
38) How do you debug an ASP.NET Core application?
Debugging is an essential skill for finding and fixing errors in your ASP.NET Core applications.
Debugging techniques using Visual Studio:
Visual Studio provides powerful debugging tools for ASP.NET Core applications. You can:
- Set breakpoints to pause execution and inspect variables.
- Step through code line by line.
- Watch variables and expressions.
- Inspect the call stack.
- Analyze memory usage and performance.
Logging errors and troubleshooting middleware:
Logging is a valuable tool for debugging and troubleshooting. You can configure logging to write to various destinations, such as the console, files, and databases. Logging errors and other events can help you identify the source of problems and understand the flow of your application.
39) What are global exception filters, and how do you use them?
Global exception filters allow you to handle exceptions globally across your application. They provide a centralized mechanism for catching and handling exceptions before they are propagated to the exception handling middleware.
Purpose of global filters for error handling:
Global exception filters can be used to:
- Log exceptions.
- Provide custom error responses.
- Perform cleanup tasks.
- Redirect users to error pages.
Customizing global error responses:
You can customize the error response that is returned to the client by implementing the IExceptionFilter interface and overriding the OnException() method.
Example global exception filter:
C#
public class GlobalExceptionFilter : IExceptionFilter
{
private readonly IWebHostEnvironment _env;
private readonly ILogger<GlobalExceptionFilter> _logger;
public GlobalExceptionFilter(IWebHostEnvironment env, ILogger<GlobalExceptionFilter> logger)
{
_env = env;
_logger = logger;
}
public void OnException(ExceptionContext context)
{
_logger.LogError(context.Exception, “Global exception occurred.”);
if (_env.IsDevelopment())
{
context.Result = new ObjectResult(new
{
error = context.Exception.Message,
stackTrace = context.Exception.StackTrace
})
{
StatusCode = 500
};
}
else
{
context.Result = new ObjectResult(new { error = “An error occurred.” })
{
StatusCode = 500
};
}
}
}
40) How do you use logging in ASP.NET Core?
Logging is an essential part of building and maintaining ASP.NET Core applications. It provides a way to record events and information about your application’s execution.
Built-in logging providers:
ASP.NET Core has built-in support for logging to various destinations, including:
Console
Debug
EventSource
EventLog
Azure App Service
Configuring log levels and custom logging:
You can configure logging in the ConfigureLogging() method of the Program class. You can specify the minimum log level, add custom logging providers, and configure log filtering.
Example logging configuration:
C#
public static IHostBuilder CreateHostBuilder(string[] args) =>
Host.CreateDefaultBuilder(args)
.ConfigureLogging(logging =>
{
logging.ClearProviders();
logging.AddConsole();
logging.AddDebug();
// Add other logging providers as needed.
})
.ConfigureWebHostDefaults(webBuilder =>
{
webBuilder.UseStartup<Startup>();
});
After testing and debugging, we’ll provide tips to help you prepare better for ASP.NET Core interviews.
ASP.NET Core Interview Preparation Tips
Here, you’ll find practical tips to streamline your ASP.NET Core interview process. These tips will help you choose the best candidates efficiently.
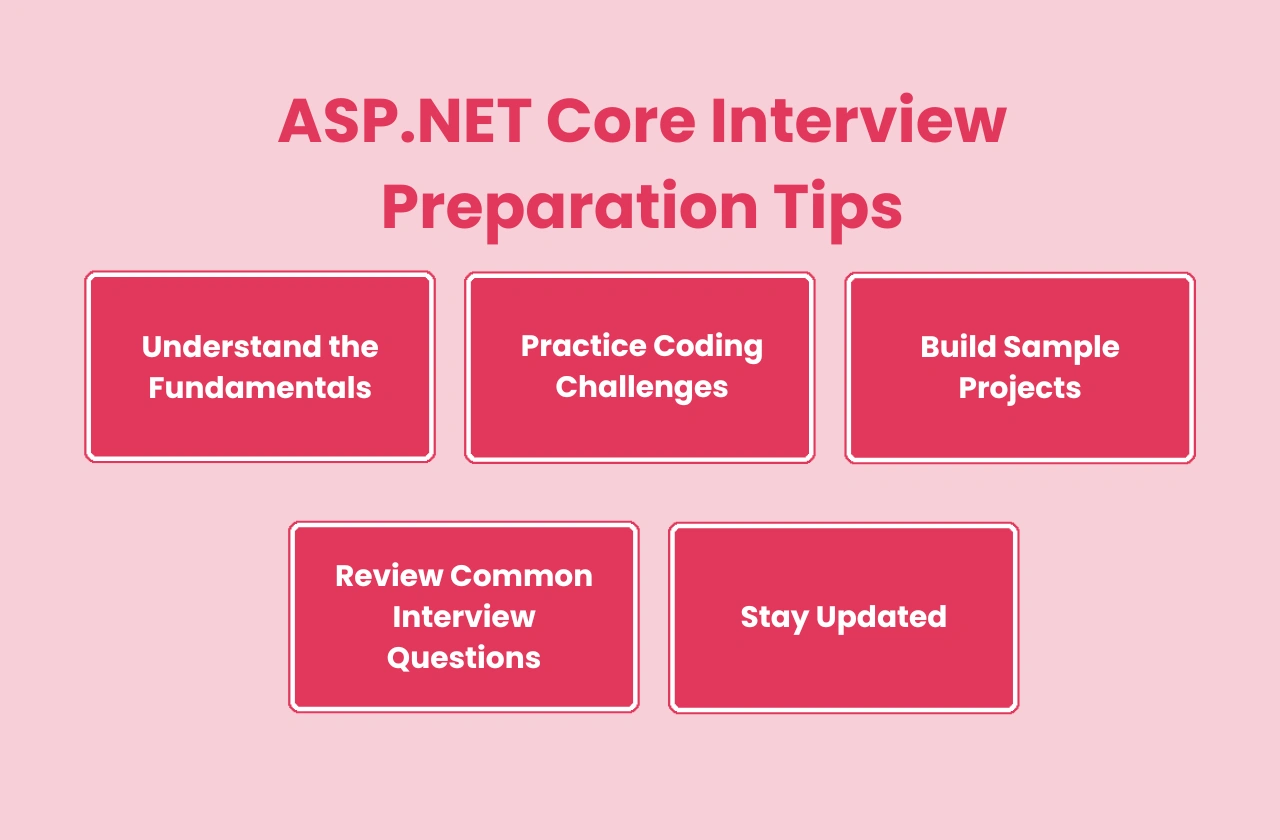
1) Understand the Fundamentals
Start with a solid understanding of the core concepts of ASP.NET Core, including:
- Request processing pipeline: How requests flow through middleware components.
- Dependency injection: How to register and inject services.
- Configuration: How to load settings from different sources.
- Routing: How to map URLs to controllers and actions.
- Model binding and validation: How to handle user input.
2) Practice Coding Challenges
Practice solving coding challenges related to ASP.NET Core. This will help you solidify your understanding of the framework and prepare you for technical interviews.
3) Build Sample Projects
Build small sample projects that demonstrate your knowledge of ASP.NET Core. This will give you hands-on experience and help you showcase your skills to potential employers.
4) Review Common Interview Questions
Review common ASP.NET Core interview questions and practice your answers. This will help you feel more confident and prepared during the interview.
5) Stay Updated
ASP.NET Core is a constantly evolving framework. Stay updated with the latest releases and features by reading blogs, articles, and documentation.
Finally, let’s wrap up with a conclusion to summarize the key points of this article.
Conclusion
This comprehensive guide has equipped you with the knowledge and tools to confidently tackle ASP.NET Core interview questions. Remember to practice your answers, build sample projects, and stay updated with the latest developments in the framework. With thorough preparation and a clear understanding of the concepts, you’ll be well-positioned to succeed in your ASP.NET Core interviews and land your dream job.