You are preparing for a coding interview at Accenture. You are looking for practice questions and answers.
We understand this is a stressful time. Technical interviews can be challenging. You want to feel confident and prepared.
This article is here to help you. We have compiled a list of common Accenture coding questions with detailed answers. We hope this resource will give you the practice you need to succeed in your interview.
Accenture’s Coding Interviews
Accenture’s coding interviews test your technical skills and how you think. They want to know if you can solve problems and code well. This guide helps you get ready.
The Interview Format
Phone Screens: Accenture often starts with a phone interview. A recruiter or engineer talks to you. They ask about your experience and skills. They might give you a simple coding problem to solve.
Virtual/Onsite Rounds: If you do well on the phone, you go to the next step. This can be online or in person. You usually have multiple interviews. Each interview lasts about an hour. You talk to different people.
What to Expect: These interviews are technical. You get coding questions. You write code on a computer or whiteboard. Sometimes, they give you a problem and ask you to talk through your solution.
Assessment Areas
Problem-solving: Accenture searches for people who can solve problems. They give you coding problems to test this. They want to see how you think and find answers.
Algorithm Design: You need to know about algorithms. These are steps to solve problems. They want to know if you can choose the right algorithm and use it.
Data Structures: You need to know how to store and organise data. This is important for coding. They might ask you to use different data structures like arrays or lists.
Coding Efficiency: Your code needs to work well. It shouldn’t use too much time or memory. They might ask you to make your code faster or smaller.
Communication: You need to talk about your code clearly. You need to explain what you are doing and why. They want to know if you can work with a team.
Programming Languages
Accenture uses different programming languages. But some are more common than others. Here are a few you should know:
- Java: This is a popular language for many things.
- Python: This language is easy to learn and use. It’s good for data science and machine learning.
- C++: This language is fast and powerful. It’s good for games and system software.
Other Languages: You might see other languages too. It depends on the job. They might ask about your favourite language. Be ready to talk about why you like it.
Now that you know what Accenture looks for, let’s see the types of questions they ask.
Accenture Coding Questions: Categories
Accenture coding interviews test your technical skills across different areas. Understanding these categories helps you prepare effectively.
Warm-Up Questions (Easy):
These questions check your grasp of basic programming concepts. They often involve:
- Loops (for, while)
- Conditional statements (if, else)
- Basic data types (integers, strings)
- Simple functions
Examples:
- FizzBuzz: Print numbers from 1 to n, replacing multiples of 3 with “Fizz”, multiples of 5 with “Buzz”, and multiples of both with “FizzBuzz”.
- Palindrome Check: Determine if a word or phrase reads the same backward as forward.
- String Manipulation: Reverse a string, count characters, find substrings.
Data Structures and Algorithms (Medium):
This category tests your knowledge of common data structures and how to use them. You might encounter:
- Arrays
- Linked Lists
- Stacks
- Queues
- Trees
- Graphs
Examples:
- Array Sorting: Implement sorting algorithms like bubble sort, insertion sort, or merge sort.
- Binary Search: Find a target value in a sorted array.
- Linked List Reversal: Reverse the order of nodes in a linked list.
- Tree Traversal: Visit all nodes in a tree (preorder, inorder, or postorder).
Problem-Solving (Medium to Hard):
These questions require you to apply problem-solving techniques to complex scenarios. Common topics include:
- Dynamic Programming: Break down problems into smaller subproblems and store solutions.
- Greedy Algorithms: Make locally optimal choices at each step.
- Backtracking: Explore all possible solutions by trying different paths and undoing choices when necessary.
Examples:
- Knapsack Problem: Choose items to maximise value within a limited weight capacity.
- Coin Change: Find the minimum number of coins needed to make a certain amount.
- String Permutations: Generate all possible rearrangements of a string.
Object-Oriented Programming (OOP) (Medium):
OOP questions assess your understanding of object-oriented principles:
- Class Design: Create classes to model real-world objects or concepts.
- Inheritance: Establish relationships between classes (parent-child).
- Polymorphism: Use a single interface to represent different types.
- Encapsulation: Bundle data and methods within a class, hiding internal details.
Examples:
- Design a Class Hierarchy: Create classes for animals, with subclasses for mammals, birds, etc.
- Implement Interfaces: Define interfaces for shapes, then create classes for circles, squares, etc., that implement these interfaces.
System Design (Hard):
System design questions test your ability to design scalable and efficient systems. You’ll need to:
- Understand the requirements of the system.
- Choose appropriate technologies and components.
- Consider scalability, performance, and fault tolerance.
- Discuss trade-offs and justify your design choices.
Examples:
- Design a URL Shortening Service: Create a system that shortens long URLs and redirects users.
- Design a Chat Application: Build a system that allows users to send messages in real-time.
- Design a Recommendation System (if relevant): Develop a system that suggests items to users based on their preferences and behaviour.
We’ve seen the question types. Let’s look at some real examples and their answers.
Accenture Coding Questions with Answers
Here are some questions Accenture asks, with answers to help you practise.
Fundamentals
1) Palindrome Check: Write a function to determine if a given string is a palindrome (reads the same backward as forward).
Python
def isPalindrome(s):
return s == s[::-1]
2) Fibonacci Sequence: Generate the first ‘n’ numbers in the Fibonacci sequence.
Python
def fibonacci(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
3) Factorial Calculation: Implement a function to calculate the factorial of a number.
Python
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n – 1)
Data Structures
4) Array Reversal: Reverse an array in place.
Python
def reverseArray(arr):
start, end = 0, len(arr) – 1
while start < end:
arr[start], arr[end] = arr[end], arr[start]
start += 1
end -= 1
5) Linked List Traversal: Traverse a linked list and print its elements.
Python
def traverseLinkedList(head):
current = head
while current:
print(current.val)
current = current.next
6) Binary Search Tree Insertion: Insert a node into a binary search tree.
Python
def insertBST(root, key):
if root is None:
return Node(key)
if key < root.key:
root.left = insertBST(root.left, key)
else:
root.right = insertBST(root.right, key)
return root
Algorithms
7) String Anagram Check: Check if two strings are anagrams (contain the same characters but in different orders).
Python
def isAnagram(s1, s2):
return sorted(s1) == sorted(s2)
8) Sorting Algorithms: Implement bubble sort, insertion sort, or merge sort.
Python
# Example: Insertion Sort
def insertionSort(arr):
for i in range(1, len(arr)):
key = arr[i]
j = i – 1
while j >= 0 and key < arr[j]:
arr[j + 1] = arr[j]
j -= 1
arr[j + 1] = key
9) Searching Algorithms: Implement linear search or binary search.
Python
# Example: Linear Search
def linearSearch(arr, target):
for i, num in enumerate(arr):
if num == target:
return i
return -1
You’ve seen some practice questions. Here are tips to do well in your interview.
Tips for Accenture Coding Interview Success
Accenture’s coding interviews test your technical skills and problem-solving ability. With the right preparation, you can increase your chances of success.
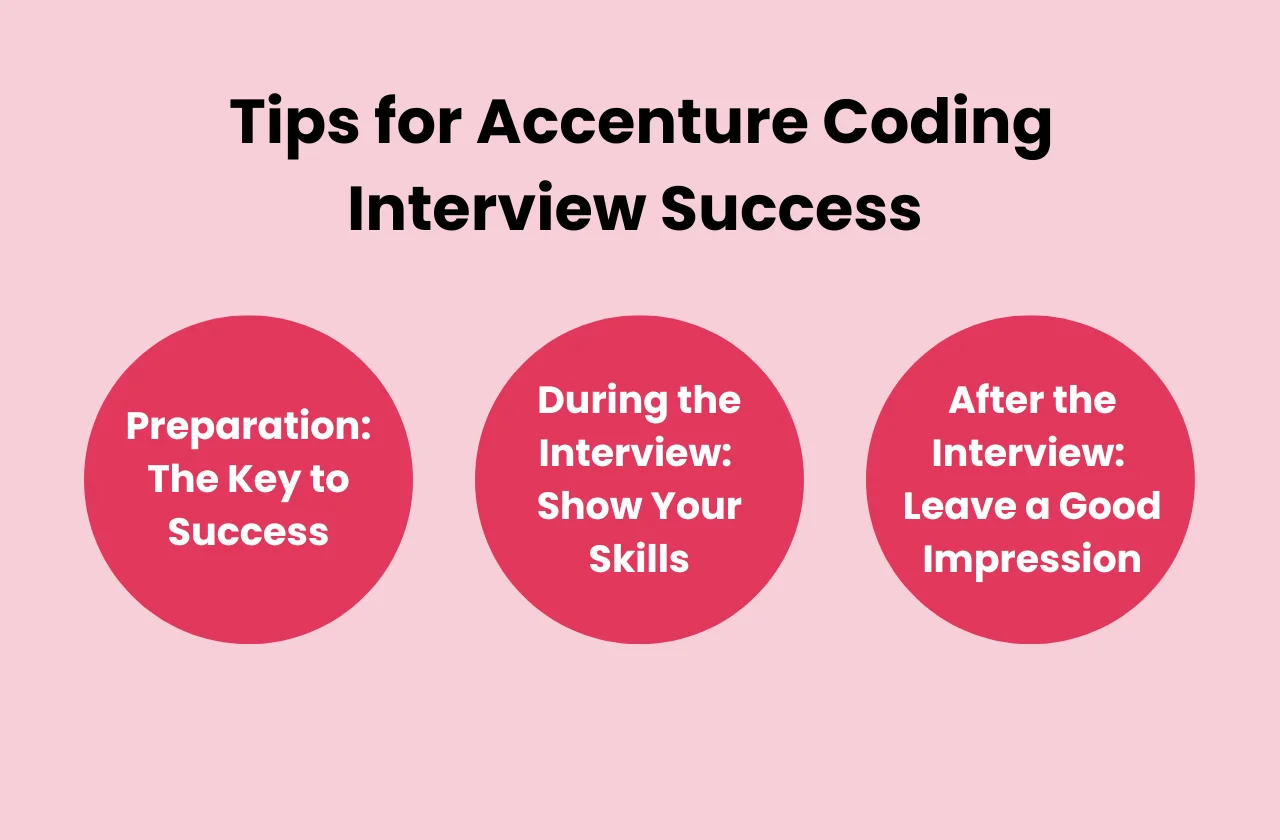
Preparation: The Key to Success
- Practice Coding Regularly: Use platforms like LeetCode and HackerRank to solve coding problems frequently. This will help you get familiar with common patterns and improve your coding speed.
- Learn the Basics of Data Structures and Algorithms: Understand how different data structures work (arrays, linked lists, trees, etc.) and common algorithms (sorting, searching, etc.). These are the building blocks for most coding problems.
- Take Mock Interviews (Use iScalePro): Simulate the interview experience with a friend or mentor. This will help you manage your time effectively and get feedback on your performance.
During the Interview: Show Your Skills
- Understand the Problem: Ask questions to clarify the problem’s requirements. Make sure you know what the expected input and output are.
- Think Out Loud: Explain your thought process as you solve the problem. This helps the interviewer understand how you approach problems.
- Write Clean Code: Use clear variable names and comments. Make your code easy to read and understand.
- Make Your Code Efficient: Consider different approaches and choose the most efficient one. Optimise your code for time and space complexity.
- Test Your Code: Check your code with different test cases, including edge cases. Make sure it works as expected.
After the Interview: Leave a Good Impression
- Say Thank You: Send a thank-you email to the interviewer. This shows your appreciation for their time.
- Express Your Interest: Reiterate your interest in the position and the company. This can help you stand out from other candidates.
Conclusion
Accenture coding questions are no longer a mystery. This article has helped you understand the types of questions and how to answer them. With practice, you can become comfortable with the format.
Don’t wait! Use iScalePro to practise Accenture coding questions today. Increase your chances of success and get one step closer to landing your dream job. Your future starts now.
FAQs Related to Accenture Coding Questions
1) Does Accenture ask coding questions?
Yes, Accenture does ask coding questions during the recruitment process. These questions are typically part of the technical assessment or coding round. The aim of these questions is to evaluate your problem-solving skills, coding ability, and understanding of data structures and algorithms.
2) Do questions repeat in Accenture?
While there’s no guarantee that questions will repeat exactly, it’s possible that you might encounter similar problems or variations of questions that you’ve seen before. It’s important to focus on understanding the underlying concepts and problem-solving techniques rather than memorizing specific questions.
3) What programming language does Accenture use?
Accenture uses a variety of programming languages, depending on the specific project or role. Some of the commonly used languages include Java, C++, Python, JavaScript, and C#. It’s a good idea to be proficient in at least one of these languages to increase your chances of success in the coding round.
4) Is DSA important for Accenture?
Yes, Data Structures and Algorithms (DSA) are crucial for performing well in Accenture’s coding rounds. DSA provides you with the necessary tools and techniques to solve complex problems efficiently. A strong understanding of DSA will help you write clean, optimized, and readable code.